Errors in programming are like hidden cracks in a foundation: they can cause unexpected crashes, incorrect results, or even complete system failures. Therefore, knowing how to spot and fix these errors is an essential part of becoming a successful programmer. In programming, there are several types of errors that students should understand, such as syntax errors, logic errors, run-time errors, and overflow errors. Each error can impact the behavior of a program in different ways. As a result, programmers must learn various techniques to identify and resolve these issues quickly. This skill is crucial not only for success on the AP® Computer Science Principles exam but also for long-term problem-solving skills in any coding project.
What We Review
Types of Errors in Programming
Syntax Errors
A syntax error occurs when the rules of the programming language are not followed. In other words, the code has a mistake in how it is written, making it impossible for the compiler or interpreter to understand. For example, forgetting a semicolon in Java or misspelling a keyword like publc instead of public will generate a syntax error.
Example:
- Incorrect code: System.out.println(“Hello world!”)
- Correct code: System.out.println(“Hello world!”);
- Notice that the missing semicolon leads to a syntax error. When a syntax error occurs, the program usually cannot run until it is fixed.
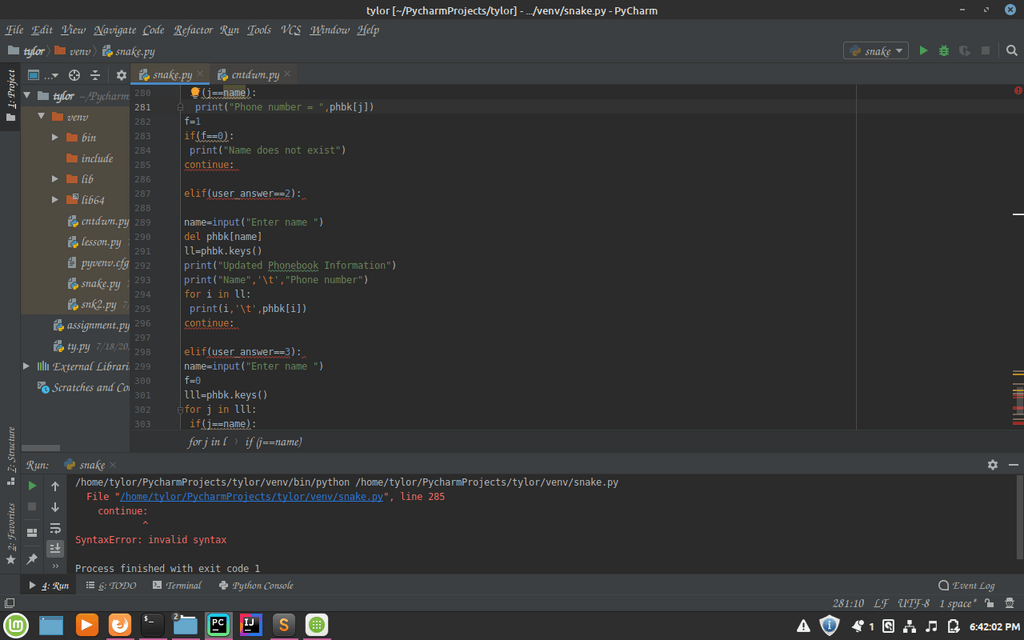
Logic Errors
A logic error is a mistake in the algorithm or program that causes it to behave incorrectly or produce the wrong output, even though the program may run without crashing. Logic errors can be tricky because the code might look correct and still compile or interpret properly. However, the outcome is not what was intended.
Example:
- Suppose there is a program that calculates the average of two numbers but accidentally subtracts them instead. It might run, but deliver the wrong answer.
- If you intended (num1 + num2) / 2, but wrote (num1 – num2) / 2, the result would make little sense for an average.
Run-time Errors
A run-time error appears while the program is executing. Even if the code seems fine at compilation, some operations can cause the application to crash or behave unexpectedly once it runs. For instance, dividing by zero triggers a run-time error, or accessing an array index that is out of range can generate an exception.
For example, assume a list of numbers is numbers ←[1, 2, 3]. Attempting to run numbers[4] will cause an error because there is no element at index 4. As a result, this causes a run-time error.
Overflow Errors
An overflow error happens when a program attempts to handle a value that exceeds the allowed range for its data type. Computers have limits on how large (or small) a number can be stored in specific variables. Therefore, any number outside the range can cause unexpected results or strange behavior.
For example, an int data type in Java typically handles values up to 2,147,483,647. Trying to store a larger number, such as 3,000,000,000, may create an overflow error and change the stored value to something unexpected.
How to Identify Errors
Testing Your Code
Testing is the first step in figuring out if a program works as intended. The best way to learn how to test code is to create multiple test cases. These test cases should include normal, extreme, and unexpected inputs. For instance, if a program sums two numbers, try using zero, negative numbers, and extremely large values. By doing this, programmers can check if the program handles all scenarios correctly. Testing helps reveal logic, run-time, or even overflow errors quickly. As a result, continuous testing during development ensures fewer issues later.
Hand Tracing
Hand tracing is a technique used to follow the flow of code manually without running it on a computer. To hand trace, write down each variable and its updated value as you “simulate” the code in your mind.
Step-by-step example:
- Write down each variable and its initial value.
- Follow each instruction in the program, updating the variable values as indicated.
- Observe any incorrect results or unexpected changes in variables. By tracing in this careful manner, logic errors often become more obvious.
Visualizations
Visualizations like flowcharts or diagrams can be especially helpful for visual learners. By mapping each step of a program, it’s easier to see how data moves through loops and conditionals. For instance, a flowchart can reveal if a loop is set up incorrectly or if a branch of logic never executes. This method is highly effective when combined with hand tracing, since the flowchart provides a big-picture view of the code’s structure and relationships.
Debuggers
Most programming tools include debuggers that allow programmers to step through code line by line, inspect variables, and pause execution at chosen points. Debuggers are extremely valuable for discovering run-time errors, such as null pointer issues or out-of-range array indices. For example, in many IDEs, setting a breakpoint on a line, running the debugger, and examining the variable state can immediately pinpoint where a program goes wrong. Using a debugger effectively saves time compared to randomly guessing the source of an error.
Extra Output Statements
Adding extra output statements is a straightforward way to see the internal program state without using a debugger. Well-placed print statements can reveal whether variables hold the correct values at different stages. For instance, inserting DISPLAY(x) inside a loop can show if a variable is updating properly. However, remember to remove or comment out these extra statements before final submission, to keep the code clean.
Strategies for Correcting Errors
After identifying the types of errors in programming, the next step is resolving them. One powerful method is looking for patterns: if the same error recurs, there might be a misunderstanding of a language feature. Another strategy is iterative testing—fix one issue, then run tests again to avoid introducing new problems inadvertently. This approach ensures that each change is verified immediately. Additionally, developers should document frequent errors and their solutions in a simple log or notebook, making repeated problems easier to address in the future. As a result, a disciplined process of identify, fix, test, and repeat will lead to more reliable and efficient code.
Practical Example: Identifying and Correcting Errors
Consider the following program, which is intended to calculate and print the average of four numbers. Notice that it contains multiple errors:
PROCEDURE AverageFinder
{
a ←10
b ← 20
c ← 30
d ← 0
a + b + c + d ← sum
average = sum / 4
DISPLAY(sum)
}
- Syntax Error – sum should be on the left side of the assignmet, sum ← a + b + c + d
- Potential Logic Error – The code displays sum instead of average. We can probably assume AverageFinder should display the average.
After fixing these issues, the corrected code would produce the right average:
PROCEDURE AverageFinder
{
a ←10
b ← 20
c ← 30
d ← 40
sum ← a + b + c + d
average = sum / 4
DISPLAY(averge)
}
By testing with different values, such as 0 or negative inputs, additional errors could be discovered early in the development process.
Quick Reference Chart of Key Vocabulary
Below is a handy list of critical terms related to identifying and correcting errors:
- Syntax Error – A mistake in the program where the rules of the language are not followed.
- Logic Error – A mistake in the algorithm or program that causes it to behave incorrectly or deliver an unexpected output.
- Run-time Error – An error that occurs during the execution of a program, such as dividing by zero.
- Overflow Error – Occurs when a program handles a number beyond its defined range.
- Test Cases – Well-defined inputs to ensure a program produces the expected outcomes.
- Hand Tracing – Manually following each step of code to find logical mistakes.
- Visualizations – Flowcharts or diagrams that illustrate how the program flows.
- Debuggers – Tools that pause execution at specific lines, allowing the programmer to inspect variable values.
- Extra Output Statements – Print statements added to reveal variable states and locate errors.
Conclusion: Types of Errors in Programming
Error identification and correction are crucial in developing robust, reliable programs. By understanding how to isolate syntax, logic, run-time, and overflow issues—common types of errors in programming—programmers can pinpoint exactly where the code fails and then swiftly fix it. Moreover, employing a variety of methods—such as testing with specific inputs, hand tracing, and using debugging tools—can transform debugging into a more structured process. In the long run, consistent error handling strengthens problem-solving skills and helps ensure successful outcomes in all programming projects.
Sharpen Your Skills for AP® Computer Science Principles
Are you preparing for the AP® Computer Science Principles test? We’ve got you covered! Try our review articles designed to help you confidently tackle real-world AP® Computer Science Principles questions. You’ll find everything you need to succeed, from quick tips to detailed strategies. Start exploring now!
- AP® Computer Science Principles 1.1 Review
- AP® Computer Science Principles 1.2 Review
- AP® Computer Science Principles 1.3 Review
Need help preparing for your AP® Computer Science Principles exam?
Albert has hundreds of AP® Computer Science Principles practice questions and full-length practice tests to try out.