Variables in programming are like labeled boxes that hold information. They make it possible to store, retrieve, and update data throughout a program. Often, data in a program changes over time, so variables help keep track of these shifting values in a structured way. Therefore, understanding how to work with variables is a major step toward writing clear, efficient code. By exploring variables, their types, how to assign them, and how their scope works, a beginner gains a powerful foundation in programming. Consequently, it becomes easier to organize projects and debug issues when variables are named well.
What We Review
What Is a Variable in Programming?
A variable in programming can be seen as a placeholder for a value. Think of it like a hotel room where guests (data) can come and go. However, the room remains the same “place,” only changing who or what occupies it. In code, a variable might store a number, a piece of text, or even a list of items. Therefore, choosing meaningful names for variables helps others easily understand what data those variables hold. For instance, score is clearer than x when representing a player’s score in a game, since it directly describes the purpose.
Types of Variables in Programming
Programming languages often provide different data types, each suited for specific situations. Here are some common examples:
- Number: Used for integers (like 5, 10, 100) or decimals (like 3.14).
- Boolean: Can only be true or false, making them essential for conditions.
- String: Represents sequences of characters, such as “Hello World”.
- List: Holds multiple values under one variable name (e.g., [2, 4, 6]).
Therefore, it is important to pick the type that best fits the data. For example, choose a Boolean if the answer is only yes/no, and select a list if many values should be stored in a single container.
Assigning a Variable
To assign a variable means to store a value in it using an assignment operator. In many languages, the symbol for assignment is =, but on the AP® CSP pseudocode sheet, the arrow ← is used. As a result, the code:
a ← 5
stores the value 5 in the variable a. The process goes like this:
- The right side of the arrow (expression) is evaluated.
- The result is placed into the variable on the left side.
Hence, line-by-line, the expression 5 is read, then a becomes 5. This allows the program to keep track of 5 under the variable name a.
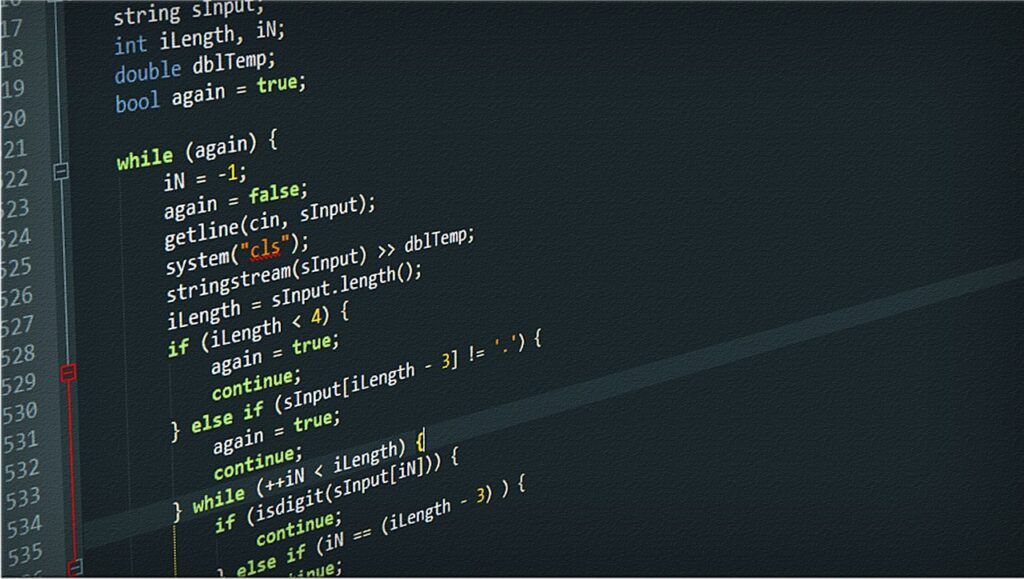
Changing Variable Values
After a variable is assigned, its value can be updated. For instance, if a was previously 5, and then the code says:
a ← 10
the variable a now holds 10. Therefore, the most recent assignment always overrides any previous value. For example:
- score ← 3
- score ← 7
In these two lines, score changes from 3 to 7. As a result, if the code prints score, the output is 7. This concept of updating allows a program to reflect new information as it runs, such as a player’s game progress.
Scope of Variables
Variable scope refers to where in the code a variable can be used or accessed. In many languages, variables can be either local or global. Local variables exist only within a specific part of a program, often inside a function or block of code. Consequently, reading or modifying them outside that block can be impossible. Global variables, however, are accessible anywhere in the program. Therefore, understanding scope matters when organizing code and preventing naming conflicts. Proper scope management also reduces unintended errors, like updating the wrong variable by mistake.
Practical Example: Step-by-Step Assignment
Imagine a simple program that tracks the number of apples in a basket:
- apples ← 5
- apples ← apples + 2
- basketMessage ← “Basket has ” + apples + ” apples.”
Here, the first line assigns 5 to apples. Next, apples is updated to its old value (5) plus 2, which makes it 7. Finally, basketMessage is assigned the string “Basket has 7 apples.” Therefore, if the program displays basketMessage, it shows exactly how many apples are in the basket at that moment.
Key Terms to Know
- Scope – The region in a program where a variable is accessible or can be used
- Algorithm – A step-by-step procedure to solve a problem
- Boolean – A data type with only two possible values: true or false
- Variable – An abstraction in a program that holds a value
- Data Type – The kind of data a variable holds, such as number, string, or Boolean
Conclusion
Variables in programming shape how data is handled and updated. They carefully store information, making it straightforward to reference or modify values over time. Therefore, selecting appropriate data types, naming variables thoughtfully, and understanding assignments all lead to cleaner code. Moreover, keeping track of which parts of the program can see each variable (scope) makes it simpler to maintain and extend code later. Practicing these concepts ensures a stronger grasp of fundamental programming ideas, ultimately laying the groundwork for success in AP® Computer Science Princples.
Sharpen Your Skills for AP® Computer Science Principles
Are you preparing for the AP® Computer Science Principles test? We’ve got you covered! Try our review articles designed to help you confidently tackle real-world AP® Computer Science Principles questions. You’ll find everything you need to succeed, from quick tips to detailed strategies. Start exploring now!
- AP® Computer Science Principles 2.1 Review
- AP® Computer Science Principles 2.2 Review
- AP® Computer Science Principles 2.3 Review
- AP® Computer Science Principles 2.4 Review
Need help preparing for your AP® Computer Science Principles exam?
Albert has hundreds of AP® Computer Science Principles practice questions and full-length practice tests to try out.