Mathematical expressions play a major role in programming and problem-solving. They form part of the instructions that tell a computer what to do and how to do it. Therefore, knowing how these expressions work is crucial in any algorithm. Algorithms are vital in computer science because they provide a finite set of steps for accomplishing a specific task. In other words, they act like roadmaps for solving problems, whether in code or in everyday life. As a result, this article explores how algorithms work, how arithmetic operators help form mathematical expressions, and why clarity matters.
What We Review
What Is an Algorithm?
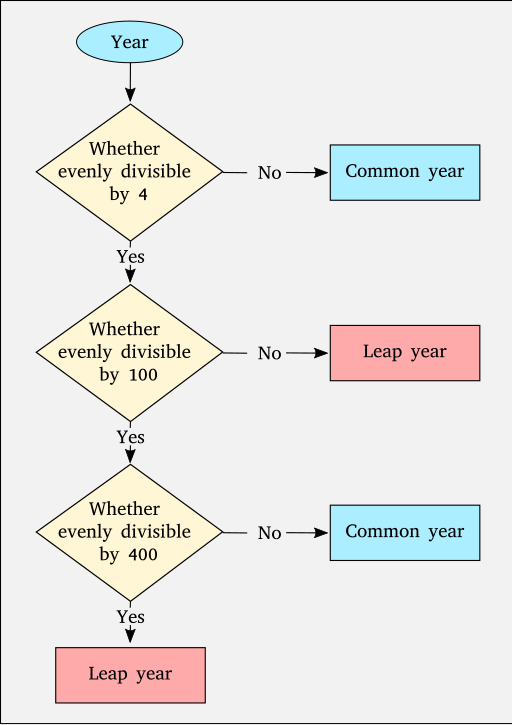
An algorithm is a finite set of instructions designed to accomplish a particular task. For example, consider the steps in a recipe that explain how to bake a cake: gather ingredients, mix them, heat the oven, then bake. Each action must happen in order, or the result may fail. Similarly, algorithms in computer science follow a sequential logic.
- Algorithms can be written in code, but they can also appear in natural language or diagrams.
- Every algorithm can be built using sequencing, selection, and iteration.
- A good algorithm ensures that each step is clear and unambiguous.
Understanding Expressions
Expressions are combinations of values, variables, operators, or procedure calls that produce a single value. They are like simple math statements that can be evaluated at any point in a program. As a result, expressions form the backbone of calculations and logic within an algorithm. For instance, 10 + 5 is an expression that evaluates to 15, while x * 2 is another expression whose result depends on the value of x.
Think of an expression like a single arithmetic problem: it has parts that come together to produce one answer. Therefore, learning how these parts fit together is crucial for writing effective algorithms.
The Role of Arithmetic Operators
Arithmetic operators are symbols that let programmers perform mathematical calculations in code. They include + (addition), – (subtraction), * (multiplication), / (division), and MOD (the mod operator). These operators act like the gears of a machine: they drive the arithmetic by taking in numbers (or variables) and producing a result.
- + adds two values together.
- – subtracts one value from another.
- * multiplies two values.
- / divides one value by another.
- MOD finds the remainder after division.
These operators are found in almost every programming language. Moreover, they help transform basic instructions into powerful algorithms that handle complex calculations.
Order of Operations
Order of operations determines the sequence in which parts of an expression are evaluated. In mathematics classes, this is often referred to as PEMDAS or BODMAS. Programming languages follow similar rules. Parentheses (or brackets) come first, then exponents, followed by multiplication and division (including MOD), and finally addition and subtraction.
For example, consider the expression 5 + 3 * 2. Multiplication takes priority, so it is computed first. As a result, the expression becomes 5 + 6, which equals 11. Failure to follow the correct order can produce unexpected results. Therefore, programmers need to be consistent and careful about how each step is evaluated.
Sequencing in Algorithms
Sequencing is executing each step of an algorithm in the order it is written. It acts like a well-organized list of tasks, ensuring that an operation is completed before moving on. In daily life, thinking about how to tie shoes demonstrates sequencing: one must first place the right lace over the left, then pull it tight, and continue step by step.
Similarly, a computer program processes code statements in order, unless directed otherwise by loops or conditionals. This structure helps answer the question, “How do algorithms work?” By breaking tasks down into clear steps, a computer can follow instructions from start to finish without confusion.
Consider a simple pseudocode representation of a recipe:
- Mix ingredients
- Heat oven
- Place mixture into oven
- Wait for 30 minutes
Each step is executed in the exact sequence shown.
Evaluating Expressions with Arithmetic Operators
Arithmetic operators allow for a range of calculations. Evaluating these expressions involves applying the order of operations to produce a single value. Below are examples that use each operator:
Single Operation Example
Expression: 5 + 3
- Add 3 to 5.
- The result is 8.
Multiple Operations Example
Expression: 5 + 3 * 2
- Multiply 3 by 2 first, resulting in 6.
- Add 5 to 6, resulting in 11.
Modulus (MOD) Example
Expression: 17 MOD 5
- Divide 17 by 5 to get 3 with a remainder of 2.
- 17 MOD 5 evaluates to 2.
Clarity and Readability in Expressions
Clear expressions are easier to maintain, debug, and share. When an expression is understandable at first glance, future developers (and you) will locate issues more quickly. Therefore, it is helpful to use parentheses to group parts of an expression that belong together. For instance, (x + 3) * 2 shows an obvious intention, even though the language might still handle the multiplication first.
Additionally, proper spacing around operators makes expressions look more organized. For example, x + 3 appears more readable than x+3. As a result, the code remains easier to interpret. These small details reduce mistakes and improve collaboration among team members.
Practice Problems
Here are a few practice problems to test understanding:
- What is the result of 20 / 4 + 2?
- Evaluate 12 MOD 5 * 3.
Example Solutions
- 20 / 4 + 2 → First do 20 / 4 = 5, then 5 + 2 = 7.
- 12 MOD 5 * 3 → 12 MOD 5 is 2, then 2 * 3 is 6.
Key Terms to Know
- Algorithm – A finite set of instructions that accomplish a specific task
- Arithmetic Operators – Symbols (+, -, *, /, MOD) that perform math operations
- Expression – A value, variable, operator, or procedure call that produces a single value
- Sequencing – Executing each step of an algorithm in the order it appears
- Code Statement – A portion of code that expresses an action
- Mod Operator – Returns the remainder after dividing one number by another
Conclusion
Mathematical expressions and algorithms are fundamental to AP® Computer Science Principles. They allow complex ideas to be expressed in a systematic, organized way. Moreover, they help answer the question of how algorithms work by providing a set of steps that execute in a predictable sequence. Understanding the flow of an algorithm and the structure of expressions ensures the code runs as intended. Above all, clarity and readability remain vital for long-term success. Continual practice with arithmetic operators, sequencing, and expression evaluation will build a solid foundation for programming in any language.
Sharpen Your Skills for AP® Computer Science Principles
Are you preparing for the AP® Computer Science Principles test? We’ve got you covered! Try our review articles designed to help you confidently tackle real-world AP® Computer Science Principles questions. You’ll find everything you need to succeed, from quick tips to detailed strategies. Start exploring now!
Need help preparing for your AP® Computer Science Principles exam?
Albert has hundreds of AP® Computer Science Principles practice questions and full-length practice tests to try out.