Algorithm development is a core skill in computer science. An algorithm is essentially a method or set of instructions for solving a particular problem. It can be as simple as following a list of steps to bake cookies or as complex as programming a self-driving car. However, in every case, the goal remains the same: transform certain inputs into desired outputs through a clear, step-by-step process. Therefore, understanding how algorithms work is essential for anyone interested in computing. This article explores how different algorithms can achieve similar results, how side effects may differ, and how new algorithms can be built or modified from existing ones. As a result, AP® CSP students can gain confidence in algorithm development and enhance their problem-solving skills.
What We Review
What is an Algorithm?
An algorithm is a carefully structured set of instructions designed to accomplish a task. Think of a recipe that outlines each step needed to bake a cake: gather ingredients (inputs), follow the steps (process), and produce a tasty cake (output). Therefore, the recipe acts like a real-life algorithm.
Algorithms have three main components:
- Input: The materials or data an algorithm receives (e.g., a list of numbers).
- Steps: The exact process or operations to be executed (e.g., addition, comparison).
- Output: The final result after the steps are completed (e.g., the maximum number in a list).
Essentially, computer algorithms follow the same pattern, except the steps are expressed as code or logic instead of cooking instructions, making algorithm development a crucial part of the process.
Comparing Algorithms
Different algorithms can handle the same task but use different approaches. It is often necessary to compare sort algorithms, search algorithms, or other processes to figure out which one is most efficient. As an illustration, consider two ways to find the maximum value in a list:
- Step 1: Start with a list of numbers (e.g., [3, 7, 2, 9, 5]).
- Algorithm A (Linear search):
- Initialize a variable max to the first value in the list.
- Next, go through each number in the list: if the current number is greater than max, update max.
- Finally, output max once the entire list has been checked.
- Algorithm B (Sorting and selecting):
- Sort the list into ascending order.
- The maximum value is the last element in the sorted list.
- Comparison: Both strategies produce the same final answer (maximum value). However, the sorting-based method might perform extra work to order all elements. Therefore, Algorithm A is often faster for simply finding the maximum, while Algorithm B might be useful if the list needs to be sorted anyway.
This type of algorithm comparison helps determine which approach works best in different scenarios.
Understanding Side Effects
Although some algorithms produce the same final result, they can still have different side effects. A side effect is any change that happens beyond the primary purpose of the algorithm. For instance, an algorithm might modify the original input data instead of leaving it untouched.
Consider these two ways to remove all negative numbers from a list:
- Step 1: Create a list of numbers (e.g., [−5, 3, −2, 10, 0]).
- First algorithm:
- Loop through the list.
- Whenever a negative number is found, remove it from the original list immediately.
- Second algorithm:
- Loop through the list and copy only non-negative numbers to a new list.
- Return this new list.
- Comparison: Both eliminate negatives but differ in side effects. The first algorithm permanently alters the original list. Meanwhile, the second algorithm does not touch the original list; it only creates a new version.
Therefore, it is important to account for side effects when choosing an algorithm.
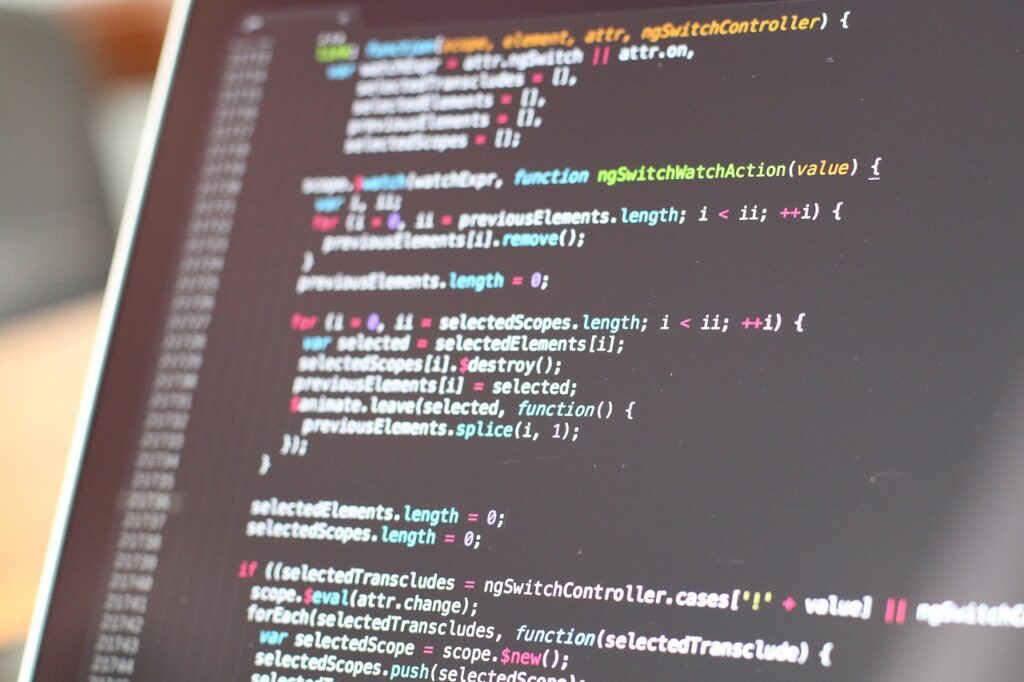
Building New Algorithms
Algorithms can be created from scratch, made by combining existing methods, or formed by modifying other algorithms. When engaging in algorithm development, it is crucial to define basic steps clearly and plan the expected result. For example, to find the average of a list of numbers:
- Step 1: Define inputs, such as a list of numbers like [2, 4, 6, 8].
- Simple algorithm for average:
- Sum up all the numbers in the list.
- Then divide the total by the count of numbers.
- Finally, return the result.
- Modification: Exclude non-numeric inputs.
- Check each item to ensure it is a valid number.
- Ignore anything that is not numeric.
- Compute the average from valid data only.
- Review: The updated algorithm filters out invalid items and calculates the average accurately.
As a result, algorithm creation often involves gradually refining existing ideas until they match the problem requirements.
Combining Algorithms
Building a complex algorithm sometimes involves splitting a problem into smaller tasks. One practical example is merging a sorting algorithm like selection sort with a maximum-finding algorithm:
- Step 1: Sort the list. Imagine sorting [5, 1, 9, 3] so that it becomes [1, 3, 5, 9].
- Step 2: Use a maximum-finding algorithm. In the sorted list, the maximum value is now the last element.
- Step 3: Combine the two steps. First, run the sorting. Next, immediately apply the maximum-finding algorithm on the sorted list.
- Step 4: Evaluate efficiency. Sorting takes more time than a simple linear scan, but it might be beneficial if sorting were required anyway.
Thus, combining and modifying existing algorithms reduces development time, simplifies testing, and often helps identify errors more quickly.
Conditional Statements and Boolean Expressions
Conditional statements control the flow of an algorithm by deciding which steps should execute based on specific conditions. Meanwhile, Boolean expressions evaluate to true or false and can be used interchangeably with certain conditional statements.
Example: Checking if a number is even:
- Step 1: Define a simple condition: “Is x even?”
- Step 2: Write the conditional statement:
- IF(x MOD 2 = 0) {DISPLAY(“x is even”)}
- Step 3: Express the same logic as a Boolean expression:
- isEven ← (x MOD 2 = 0)
- Step 4: Illustrate equivalence. If isEven is true, it matches the outcome of the conditional statement. Therefore, one can be easily replaced with the other in many algorithm structures.
As a result, learning how to rewrite conditions as Booleans (and vice versa) allows for clearer and more flexible algorithm design.
Key Terms to Know
- Algorithm – A step-by-step procedure to solve a problem
- Side Effect – An unintended change caused by an algorithm’s operation
- Conditional Statement – A statement that executes code only if a condition is met
- Boolean Expression – An expression that evaluates to either true or false
- Efficiency – A measure of how effectively an algorithm performs its intended task
Conclusion
Algorithm development lies at the heart of problem-solving in computer science. Different approaches can be used to accomplish the same tasks, and many appear similar yet yield distinct side effects. By understanding these nuances, coders can choose the best tools for each situation. Moreover, learning to create new algorithms from existing ones can save a tremendous amount of time.
Sharpen Your Skills for AP® Computer Science Principles
Are you preparing for the AP® Computer Science Principles test? We’ve got you covered! Try our review articles designed to help you confidently tackle real-world AP® Computer Science Principles questions. You’ll find everything you need to succeed, from quick tips to detailed strategies. Start exploring now!
- AP® Computer Science Principles 3.6 Review
- AP® Computer Science Principles 3.7 Review
- AP® Computer Science Principles 3.8 Review
Need help preparing for your AP® Computer Science Principles exam?
Albert has hundreds of AP® Computer Science Principles practice questions and full-length practice tests to try out.