Libraries play a major role in computer science, especially when learning to create efficient programs. In AP® Computer Science Principles, understanding what is a library in programming and how it works is essential. Consequently, exploring their features and benefits can greatly boost confidence in writing quality code.
Programming involves solving problems by writing code that tells a computer what to do. However, creating every single part of a program from scratch can be time-consuming. Therefore, programmers often use resources called libraries. These libraries offer pre-written code that can be reused in new projects. As a result, it becomes simpler to build complex applications without reinventing the wheel.
What We Review
What is a Library?
A library in programming is a collection of pre-written code, such as classes, functions, or procedures, that can be used by other programs. Thus, a software library contains procedures that may be used in creating new programs. Programmers can pick and choose from these existing code segments to accomplish common tasks more efficiently.
Libraries often come bundled with programming languages as “standard libraries,” or they can be obtained from other sources as “third-party libraries.” These shortcuts reduce the amount of code needed, thereby making it easier to build complex solutions. Above all, libraries empower programmers to focus on unique features rather than rewriting basic functionality.
Example of a Library
Imagine a toolbox stocked with tools for different tasks. In programming, a library is like that toolbox. For example, in Python, the pandas library helps with data analysis by providing helpful functions to manipulate tables and datasets.
Consider a simple Python snippet for calculating an average:
import pandas as pd
data = [10, 20, 30, 40]
series = pd.Series(data)
average = series.mean()
print("The average is:", average)
- import pandas as pd: Loads the library into the program.
- data = [10, 20, 30, 40]: Creates a list of numbers.
- series = pd.Series(data): Converts the list into a pandas structure.
- average = series.mean(): Uses a built-in pandas function to find the average.
- print(“The average is:”, average): Displays the result.
Notice how pandas simplifies complex calculations in just a few lines.
How Libraries Work
Libraries integrate seamlessly with programming languages through defined interfaces or protocols. These interfaces tell programs how to call the library’s functions and what types of data to send. In other words, libraries serve as building blocks, and developers tap into them by writing specific commands.
However, it’s not enough to simply include a library; developers must understand how the library’s components work together. Therefore, reading official documentation and exploring examples becomes crucial. By studying this information, any programmer can confidently use a library in projects.
Understanding APIs
Sometimes, libraries come with a set of rules known as an API. So, what does API stand for? API means Application Program Interface. An API explains how APIs work by detailing the procedures, classes, or endpoints that a program can call. It acts like a contract that specifies the methods available, their inputs, and their outputs.
A useful analogy is a restaurant menu. When ordering from a menu, customers choose dishes based on listed ingredients. Similarly, when using an API, developers select specific functions from the “menu” of a library. The API guarantees that those functions work exactly as described.
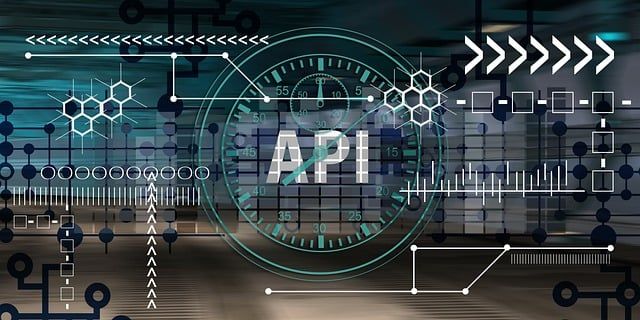
Example of an API
Suppose you want to create a weather app that fetches current conditions for a city. Many websites offer a weather API with these steps:
- Sign up for an API key to identify your app.
- Send a request using a URL like: https://api.weather.com/getWeather?city=Miami.
- Receive data in a consistent format, such as JSON.
- Extract temperature, humidity, and other details to display in your app.
Types of Libraries
There are two main types of programming libraries:
- Standard libraries: Bundled with a programming language. For instance, Java’s standard library includes built-in classes for handling input and output.
- Third-party libraries: Created by individuals or organizations outside the language’s core development team. The developer community often shares these libraries to extend a language’s capabilities.
Existing code segments can originate from internal (standard) or external (third-party) libraries. Because they streamline the programming process, libraries help create more powerful solutions with fewer bugs.
Example of Using a Third-Party Library
A popular third-party library in Python is NumPy, which is designed for numerical computations:
import numpy as np
numbers = [1, 2, 3, 4, 5]
array = np.array(numbers)
sum_value = np.sum(array)
mean_value = np.mean(array)
print("Sum is:", sum_value)
print("Mean is:", mean_value)
- import numpy as np: Loads the NumPy library.
- numbers = [1, 2, 3, 4, 5]: Prepares a list of values.
- array = np.array(numbers): Converts the list into an array for fast math operations.
- sum_value = np.sum(array): Uses NumPy’s built-in sum function.
- mean_value = np.mean(array): Calculates the average.
- print(“Sum is:”, sum_value): Shows the computed sum.
- print(“Mean is:”, mean_value): Shows the computed mean.
By relying on NumPy, programmers can avoid writing complex math functions from scratch.
Why Use Libraries?
Libraries offer numerous benefits that accelerate software development:
- Efficiency: Instead of building everything from the ground up, developers can rely on proven code that has been tested.
- Code reusability: Similar tasks do not have to be reprogrammed multiple times.
- Improved productivity: By focusing on the unique aspects of a project, programmers save time and effort, leading to faster results.
As a result, the use of libraries simplifies the task of creating complex programs. Furthermore, by leveraging existing code, teams can deliver robust software more quickly. Therefore, libraries stand as a cornerstone of modern programming.
Documentation: The Key to Understanding Libraries
Although libraries can be powerful, proper usage depends on good documentation. Documentation for an API/library is necessary to understand the behaviors provided by the API/library and how to use them. Therefore, developers should always refer to the official guides, which describe each function, its inputs, and any error messages.
Short tutorials or example snippets are often included to show how the functions behave in real-world scenarios. As a result, reading documentation can help avoid bugs. Also, it can spark ideas on how to solve tricky coding problems. Whenever encountering an unfamiliar library, spend a few minutes consulting its official references.
Example of Reading Documentation
Python has a large standard library whose documentation can be accessed online. Take the requests library in Python, commonly used for web requests. The documentation might say:
- requests.get(url, params=None): Sends a GET request to the specified URL, with optional parameters.
- .json(): Converts the response data to JSON format if available.
A simple step-by-step approach to reading this documentation is:
- Read the library’s homepage or introduction to learn its purpose.
- Look for a quick-start guide illustrating basic usage.
- Explore function-level details to understand how to call each method.
- Practice with small examples to confirm what you’ve learned.
Following this process helps you learn how to properly use any library.
Key Terms to Know
- Library – A collection of pre-written code, such as classes or functions, that can be reused in new projects.
- API – Application Program Interface; the rules describing how programs can interact with a library’s features.
- Standard Library – The built-in tools and methods that come bundled with a programming language.
- Third-Party Library – A library created by someone outside the language’s core development team, often downloaded and added to projects.
- Documentation – Written references that explain how to use and understand a library, often including examples and function details.
Conclusion: What is a Library in Programming?
A library in programming can be seen as a carefully curated toolbox that holds ready-made solutions for various tasks. It saves time, boosts creativity, and encourages collaboration. Most importantly, it teaches the value of building upon existing resources. Meanwhile, APIs guide developers in applying these libraries by laying out the rules and available functions. By learning to navigate documentation, students can plug libraries into projects effectively. Ultimately, combining libraries with original code leads to faster, more reliable programs.
Sharpen Your Skills for AP® Computer Science Principles
Are you preparing for the AP® Computer Science Principles test? We’ve got you covered! Try our review articles designed to help you confidently tackle real-world AP® Computer Science Principles questions. You’ll find everything you need to succeed, from quick tips to detailed strategies. Start exploring now!
- AP® Computer Science Principles 3.11 Review
- AP® Computer Science Principles 3.12 Review
- AP® Computer Science Principles 3.13 Review
Need help preparing for your AP® Computer Science Principles exam?
Albert has hundreds of AP® Computer Science Principles practice questions and full-length practice tests to try out.