Searching through data is a fundamental concept in computer science. When looking for a specific piece of information, it is helpful to find it quickly and efficiently. Therefore, understanding different search methods is crucial for anyone studying algorithms. One of the most efficient search methods for sorted data is binary search. Binary search eliminates large portions of data in each step. As a result, it is often faster than a simple linear search. This article explores the binary search algorithm, its requirements, how it works, and examples to show why it is so effective. The goal is to provide a clear, step-by-step explanation that is easy to follow and remember.
What We Review
What is Binary Search?
Binary search is an algorithm that begins by examining the middle element of a sorted data set. If the middle element is the desired value, the algorithm stops immediately. However, if the target value is not found, the algorithm decides which half of the data to eliminate. Therefore, binary search narrows down the search space by half after each comparison.
Imagine looking for a friend’s name in an alphabetical phone book. Instead of starting at page one, binary search has you flip to the middle page. Next, depending on whether the friend’s name comes before or after the middle entry, one only checks the relevant half. As a result, the search takes fewer flips (iterations) than going page by page.
How the Binary Search Algorithm Works
The binary search algorithm consists of:
- Finding the middle index of the sorted data set.
- Comparing the target value with the middle element.
- Determining whether the target is larger, smaller, or equal to the middle element.
- Narrowing the range of possible locations by selecting the half in which the target might lie.
- Repeating the process until the target is found or the range is empty.
To visualize, think of a line divided into equal parts, each part representing data in sorted order. The middle element is the midpoint. If the target is bigger, focus only on the right half. However, if it is smaller, concentrate on the left half. Repeat this “divide and conquer” pattern until locating the exact position of the target.
Requirements for Binary Search
Binary search depends on two main requirements:
- The data must be in a sorted order. If the data is not sorted, binary search cannot decide which half to discard.
- There must be a clear way to identify the lower and upper limits of the search. As a result, the algorithm always knows which part of the data still needs inspection.
Without these requirements, the algorithm either fails or becomes less efficient. For example, using binary search on a random list of numbers would not make sense. However, once the data is sorted, binary search becomes an excellent tool.
Number of Iterations Required
One of the greatest strengths of the binary search algorithm is its efficiency. Each step cuts the search space in half. As a result, the number of iterations grows logarithmically. In simpler terms, the number of iterations needed can often be approximated by log₂(n) for a data set of size n.
For example, if there are 16 elements, the algorithm can find (or eliminate) the target in at most 4 comparisons (because log₂(16) = 4). Meanwhile, a linear search could require up to 16 comparisons in the worst case. Therefore, binary search can save a lot of time when dealing with large data sets.
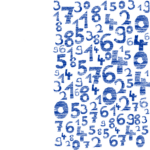
Practical Example of Binary Search
Imagine a sorted list of ages in ascending order: [13, 15, 18, 21, 24, 27, 30, 33], and the target age is 24.
- The algorithm starts by looking at the middle elements. For 8 elements, the middle can be between the 3rd and 4th positions, so it might first check the fourth element (value 21).
- Compare 21 with 24. Since 21 is less than 24, the algorithm narrows the search to the right half: [24, 27, 30, 33].
- Again, find the middle. Now the middle might be the sixth element (value 27). Compare 27 with 24. Because 27 is greater, the search narrows to [24].
- Compare 24 with 24. A match is found, so the search ends successfully.
Therefore, it took just three comparisons to locate the target value.
Code Trace Example
Using a one-based index for the list nums = [45, 54, 55, 68, 74, 90, 98] with a target of 54:
- left = 1, right = 7 → mid = 4 → nums[4] = 68 (68 > 54, so move right boundary to mid – 1).
- left = 1, right = 3 → mid = 2 → arr[2] = 54 (54 = 54, found it!).
Hence, the result is index 2.
Advantages of Binary Search
Binary search has several documented benefits:
- Speed: Thanks to its divide-and-conquer approach, each comparison halves the search space, making it ideal for large data sets.
- Predictable Performance: The time complexity is O(log n), so the search speed increases very efficiently as the data set grows.
- Straightforward Logic: The algorithm is relatively simple to implement. However, careful attention to boundary conditions is necessary.
In contrast, linear search has a worst-case time complexity of O(n), which can be significantly slower if n is large. Therefore, binary search is often preferred when the data set is sorted, and quick lookups are necessary.
Key Terms to Know
Below are some important terms and definitions related to binary search:
- Binary Search – An algorithm that begins by examining the middle of a sorted data set and discards half of the data after each comparison.
- Linear (Sequential) Search – A basic method of checking each element in order until the target is found or the end is reached.
- Middle Index – The central position in the current search range, calculated by (left + right) / 2 in many implementations.
- Logarithmic Complexity – A time complexity where the number of operations grows with log₂(n), indicating that each step halves the data set.
- Sorted Data – An arrangement of data in ascending or descending order, essential for binary search to work correctly.
- Search Space – The portion of the array still being considered, which shrinks on each iteration.
Conclusion
Binary search is a powerful tool for quickly locating items in sorted data sets. In each iteration, it precisely points to the half that might contain the target, thereby reducing the search space. As a result, fewer comparisons are required, leading to faster overall performance. Understanding the requirements for binary search, such as sorted data and defined boundaries, is vital for success in algorithm design.
Mastering this technique involves practicing with different data sets, ensuring the data is sorted, and carefully managing the algorithm’s boundaries. Therefore, learners can build confidence with repeated hands-on coding exercises. Ultimately, binary search is a cornerstone of efficient data retrieval, making it an essential skill for AP® Computer Science Principles and beyond.
Sharpen Your Skills for AP® Computer Science Principles
Are you preparing for the AP® Computer Science Principles test? We’ve got you covered! Try our review articles designed to help you confidently tackle real-world AP® Computer Science Principles questions. You’ll find everything you need to succeed, from quick tips to detailed strategies. Start exploring now!
- AP® Computer Science Principles 3.8 Review
- AP® Computer Science Principles 3.9 Review
- AP® Computer Science Principles 3.10 Review
Need help preparing for your AP® Computer Science Principles exam?
Albert has hundreds of AP® Computer Science Principles practice questions and full-length practice tests to try out.