Iteration in programming is crucial for solving repetitive tasks. It allows an algorithm to cycle through a set of instructions multiple times. Therefore, it helps create effective solutions for tasks such as counting, searching, and summarizing data. This article explores what iteration is, how it works in pseudocode, and how to avoid common iteration pitfalls. Moreover, it demonstrates the significance of iteration in everyday tasks, both in and out of programming. By focusing on the REPEAT n TIMES and REPEAT UNTIL(condition) statements provided by the AP® Computer Science Principles exam reference sheet, the discussion covers essential knowledge needed to excel in iteration-related questions.
What We Review
What Is Iteration in Programming?
Iteration refers to repeating a portion of an algorithm until a certain number of repetitions have occurred or a specific condition has been met. In simpler terms, it is like running laps around a track. Each lap represents a single pass through the repeated instructions.
Consider a race track analogy: the runner (the program) continuously goes around the track (the set of instructions) until reaching a final goal. However, the reason for continuing or stopping may vary. Sometimes, the runner completes a set number of laps (for example, 5 laps), while other times, the runner loops around until a certain signal says stop.
Iteration is fundamental in computer science since many problems require doing the same steps repeatedly. Therefore, a firm grasp of iteration helps build strong algorithmic solutions.
Types of Iteration Statements
Iteration statements, often called loops, come in different forms. Two common varieties are REPEAT n TIMES and REPEAT UNTIL(condition). Each type controls how many times the loop body runs.
REPEAT n TIMES
REPEAT n TIMES is used to execute a block of statements exactly n times. The variable n represents how many times the loop body will run. Therefore, this statement is especially handy when the number of repetitions is already known.
Example Scenario: Counting From 1 to 5
- Imagine wanting to print the numbers 1 through 5. One could write:
x←1
REPEAT 5 TIMES
{
DISPLAY(x)
x ← x + 1
}
Step-by-step breakdown:
- First repetition: Print “1”
- Second repetition: Print “2”
- Third repetition: Print “3”
- Fourth repetition: Print “4”
- Fifth repetition: Print “5”
This approach ensures the loop completes exactly five passes. As a result, it eliminates guesswork about when to stop.
REPEAT UNTIL(condition)
REPEAT UNTIL(condition) is used when the number of repetitions is unknown. The condition is checked before each loop iteration. Hence, the loop continues until the condition becomes true.
Example Scenario: Asking for Valid User Input
- Suppose a program wants to keep asking a user for a number between 1 and 10. The pseudocode might look like this:
guess ← false
REPEAT UNTIL(guess = true)
{
number ←INPUT()
IF(number = 6)
{
guess ← true
}
}
In REPEAT UNTIL(condition) loops, the algorithm keeps trying. However, if the condition is already true at the start, the loop body does not execute at all.
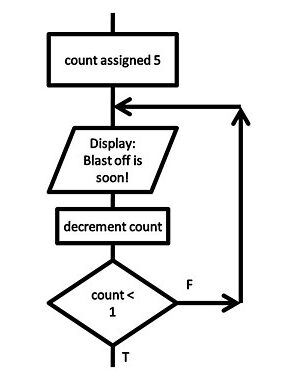
Common Pitfalls of Iteration
Iteration can lead to glitches if not handled properly. One of the most frequent issues is an infinite loop. This happens when the stopping condition never becomes true, causing the loop to repeat forever.
Example Scenario: In a REPEAT UNTIL(condition) loop, failing to change the variables inside the loop might cause the condition never to become true. Consequently, the program gets stuck.
Step-by-step analysis:
- The condition is checked. If still false, the loop continues.
- The condition remains false because no updates occur in each iteration.
- The loop never ends, resulting in a frozen or crashed program.
It is critical to confirm that the loop updates its counters or variables so the condition can eventually change to true.
Analyzing Iteration Statements
Consider the pseudocode:
sum ← 0
REPEAT 5 TIMES
{
sum ← sum + 2
}
Step-by-step explanation:
- The variable sum starts at 0.
- In each iteration, 2 is added to sum.
- After the first repetition, sum = 2.
- After the second repetition, sum = 4.
- It continues until the fifth repetition, ending with sum = 10.
Therefore, before coding, it is helpful to simulate each loop pass. This method reveals the final value or indicates whether a loop may run forever.
Real-World Applications of Iteration
Iteration is everywhere in computer science. For instance, looping in computer programming appears in:
- Game Development: Characters move continuously, scanning the environment in each loop to decide the next action.
- Data Analysis: A script may loop through thousands of data entries, adding or filtering records based on certain conditions.
- Website Navigation: Pages often refresh or update repeatedly to show dynamic content, such as live news feeds.
Moreover, iteration helps reduce redundant work and makes algorithms more efficient. As a result, programmers can perform tasks rapidly, even with vast amounts of data. Learning what iteration in programming accomplishes enriches problem-solving skills and boosts creativity.
Practice Problems
Below are some practice questions designed to reinforce iteration concepts.
1. Counting Down from 5
- Write a REPEAT n TIMES loop that prints 5 down to 1.
- Evaluate how many times the loop runs and what it prints.
- Verify correctness by tracing the loop step by step.
2. Guessing Game
- Use REPEAT UNTIL to keep asking a user to guess a secret number until the user guesses correctly.
- Show a step-by-step trace for the scenario where the user guesses wrong twice before finally guessing correctly.
- Identify what happens if the user guesses the secret number immediately.
3. Calculating a Running Total
- Initialize a variable total to 0.
- Write pseudocode using REPEAT UNTIL(condition) to add an incoming list of numbers until reading a sentinel value (e.g., -1).
- Explain how to avoid an infinite loop if the sentinel never appears.
Key Terms to Know
- Iteration – Repeating a portion of an algorithm multiple times
- Looping – Executing a block of code repeatedly until a stopping condition
- REPEAT n TIMES – Executes a block of code exactly n times
- REPEAT UNTIL(condition) – Repeats a block of code until the condition is true
- Infinite Loop – A loop that never ends due to an unmet terminating condition
Conclusion
Iteration in programming is a vital skill that opens the door to more efficient and concise code. Understanding how to use both REPEAT n TIMES and REPEAT UNTIL(condition) statements avoids tricky infinite loops. Furthermore, mastering iteration ensures the ability to analyze, troubleshoot, and optimize algorithms. It appears in numerous real-world applications, such as games, data processing, and interactive web pages.
There are many ways to practice and explore these looping constructs. Consistent application of iteration in programming helps develop deeper problem-solving techniques and builds confidence. Therefore, continued practice with the provided examples and relevant exercises strengthens intuition and aligns well with the AP® Computer Science Principles exam requirements.
Sharpen Your Skills for AP® Computer Science Principles
Are you preparing for the AP® Computer Science Principles test? We’ve got you covered! Try our review articles designed to help you confidently tackle real-world AP® Computer Science Principles questions. You’ll find everything you need to succeed, from quick tips to detailed strategies. Start exploring now!
- AP® Computer Science Principles 3.5 Review
- AP® Computer Science Principles 3.6 Review
- AP® Computer Science Principles 3.7 Review
Need help preparing for your AP® Computer Science Principles exam?
Albert has hundreds of AP® Computer Science Principles practice questions and full-length practice tests to try out.