Conditionals form the backbone of decision-making in programming. They enable a program to choose different paths based on specific conditions. For example, a simple program might check if a user’s login credentials are correct and then either grant or deny access. However, some situations call for more complex decisions. That’s where nested conditionals become crucial. Nested conditionals help handle multiple layers of checks within the same code block, which is especially useful in real-world applications like grading systems or game logic. Therefore, understanding nested conditionals leads to more flexible programs. This article explores the basics of conditionals, how to nest them, and why they are vital for systematic problem-solving.
What We Review
What are Conditionals?
Conditionals are statements in code that help decide what happens next. In other words, they check a condition (such as whether a number is greater than ten) and run a certain block of code if that condition is true. If the condition is false, they can run a different block of code instead. The simplest form of a conditional is often called an if-else statement, which checks one condition and provides an alternate path when that condition fails.
Moreover, conditionals bring logic to code by precisely controlling the flow of a program. They make it possible to handle different scenarios, such as verifying passwords or deciding if a user is old enough to purchase certain items.
Example: Simple Conditional
Imagine a program that checks whether you passed a test:
IF(score >= 60)
{
DISPLAY("You passed!")
}
ELSE
{
DISPLAY("Keep trying!")
}
- First, the code compares the value of score to 60.
- Next, if the score is 60 or higher, the program prints “You passed!”
- Otherwise, it prints “Keep trying!”
This basic structure shows how conditionals guide a program based on true-or-false checks.
What are Nested Conditionals?
Nested conditionals happen when one conditional statement exists inside another. Sometimes, a single check isn’t enough. One might need to check whether someone passed the test in one conditional, and then check if they earned an extra reward in another conditional found inside the first. This layering is what makes nested if condition structures so useful.
However, nested conditionals can also become tricky. Each inner conditional only runs if its outer condition is true. As a result, the flow becomes more complex. Nonetheless, this complexity allows for more detailed decision-making. Programmers often rely on nested conditionals to handle scenarios involving multiple criteria, such as time of day, user roles, or even specific error codes.
Example: Nested Conditional
Consider the following code:
IF(score >= 60)
{
IF(score >= 90)
{
DISPLAY("You passed with distinction!")
}
ELSE
{
DISPLAY("You passed!")
}
}
ELSE
{
DISPLAY("Keep trying!")
}
- First, the outer if checks if score is at least 60.
- Then, if that’s true, it enters the inner if to check if score is 90 or above.
- Finally, if it’s 90 or above, the program prints “You passed with distinction!” Otherwise, it prints “You passed!”
Visualizing Nested Conditionals
It often helps to visualize each step in a flowchart. A flowchart provides a diagram of choices that branch off based on true or false answers. Therefore, when code contains multiple layers, flowcharts keep track of exactly which path is taken.
Flowcharts use shapes like diamonds for decisions and arrows for flow direction. For nested conditionals, one diamond might lead into another diamond, showing each layer of logic.
Example: Flowchart Creation
- Draw a diamond labeled “score >= 60?”
- If true, draw a second diamond labeled “score >= 90?”
- If true, draw a box that says “You passed with distinction!”
- If false, draw a box that says “You passed!”
- If the first diamond is false, draw a box that says “Keep trying!”
Through this process, the branching decisions become clearer, ensuring that each path is both visible and easy to trace.
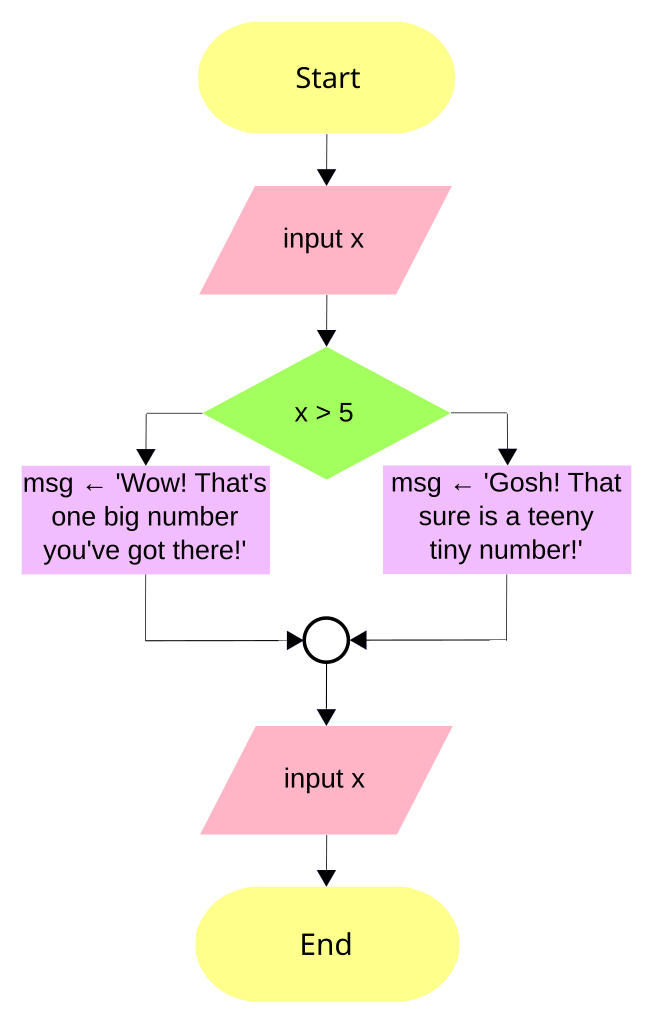
Practical Applications of Nested Conditionals
Nested conditionals appear in many real-world scenarios. For instance, a login page might check if a user is registered (outer condition) and then check if the user’s password is correct (inner condition). Similarly, games often ask multiple questions, such as whether a character has enough points to level up and if a bonus condition is met. In school grading systems, nested conditionals help assign different letter grades, then apply extra rewards for top scorers.
Using nested conditionals effectively allows programs to handle a series of checks without bulky, repetitive code. Each layer precisely targets a particular condition, making the logic both clean and adaptable.
Example: Grading System
Consider a scenario where a teacher wants to award an “A+” to students scoring above 95:
IF(score >= 60)
{
IF(score >= 90)
{
IF(score > 95)
{
DISPLAY("A+ grade");
}
ELSE
{
DISPLAY("A grade")
}
}
ELSE
{
DISPLAY("Regular pass")
}
}
ELSE
{
DISPLAY("Fail")
}
Each nested layer checks progressively more specific conditions, enabling precise outcomes.
Troubleshooting Nested Conditionals
Nested conditionals can be confusing if not managed properly. Therefore, it’s important to keep track of matching braces and ensure the correct parts of the code execute. Common errors include missing a curly brace, mixing up else statements with the wrong if, and placing code in the wrong block. As a result, the program might behave unpredictably or fail to run.
When debugging nested conditionals, consider these steps:
- Carefully indent code to visually separate each level.
- Use print statements inside each block to see which conditions are being met.
- Revisit the logic to confirm the hierarchy of decisions.
With these tips, writing effective nested if condition statements becomes easier over time.
Summary of Key Points
- Conditionals allow programs to make decisions based on whether a statement is true or false.
- Nested conditionals embed one decision structure within another to handle complex logic.
- Flowcharts help visualize how each branch leads to a different outcome.
- In practical applications, nested conditionals streamline checks in systems like grading or authentication.
- Debugging calls for careful code organization, proper use of braces, and clear print statements to track the program’s path.
Key Terms to Know
- Algorithm – A step-by-step procedure to solve a problem
- Conditional – A statement that checks whether a condition is true or false
- Nested Conditionals – Conditional statements within conditional statements
- Boolean – A data type with only two possible values: true or false
- Flowchart – A visual representation of sequential and conditional processes
Conclusion
Nested conditionals provide a robust way to manage multiple decisions in a single block of code. Therefore, it is essential to master this concept to write programs that can handle complex tasks, such as advanced user authentication or elaborate grading policies. As a result, practicing nested if condition statements helps build strong problem-solving skills. After reading this article, explore new examples and create flowcharts to visualize the logic. Finally, keep testing different scenarios to gain confidence with nested conditionals and discover the power of well-structured code.
Sharpen Your Skills for AP® Computer Science Principles
Are you preparing for the AP® Computer Science Principles test? We’ve got you covered! Try our review articles designed to help you confidently tackle real-world AP® Computer Science Principles questions. You’ll find everything you need to succeed, from quick tips to detailed strategies. Start exploring now!
- AP® Computer Science Principles 3.4 Review
- AP® Computer Science Principles 3.5 Review
- AP® Computer Science Principles 3.6 Review
Need help preparing for your AP® Computer Science Principles exam?
Albert has hundreds of AP® Computer Science Principles practice questions and full-length practice tests to try out.