Lists are one of the most important tools in programming. They allow programmers to store and organize multiple pieces of data in an ordered way. Furthermore, they make it easier to manage collections of items. For example, think of a shopping checklist that keeps track of everything needed at the grocery store. Just as it helps organize errands, this structure in computer science helps organize data. Understanding what is a list is essential in AP® Computer Science Principles, as learning how to work with lists sets a strong foundation for more advanced programming and algorithm development.
What We Review
What Is a List?
A list is an abstract data type meant to hold a sequence of elements. Each element has a specific position, often called an index. For instance, a shopping checklist could place “eggs” at index 0, “milk” at index 1, and “bread” at index 2. By treating the collection as this data structure, a program can access any element quickly. A physical shopping checklist is easy to modify by adding or crossing off items. Likewise, in programming, this type of structure can be changed with operations such as inserting, removing, or updating elements.
Basic List Operations
Mastering list operations is crucial for success in programming. These include accessing elements, assigning values, and calling specific procedures. By knowing these basics, it becomes simpler to build algorithms that traverse or modify data.
Accessing Elements
One of the most common tasks with this data structure is accessing an element by its index. In AP® Computer Science Principles, lists start at index 1.
Example:
Code Snippet:
fruits ← ["apple", "banana", "cherry"]
DISPLAY(fruits[1])
Step-by-Step:
- The list named fruits is set to hold three elements.
- The statement fruits[1] looks at the item in the first position.
- The output prints “apple.”
Assigning Values
Besides accessing information, it is useful to know how to assign a value to a particular index in a list. Assigning a value replaces whatever existed at that position.
Example:
Code Snippet:
numbers ← [10, 20, 30]
numbers[1] ← 25
Step-by-Step:
- The list named numbers has three elements: 10, 20, and 30.
- The statement numbers[1] ← 25 updates the first element from 10 to 25.
- Now, numbers is [25, 20, 30].
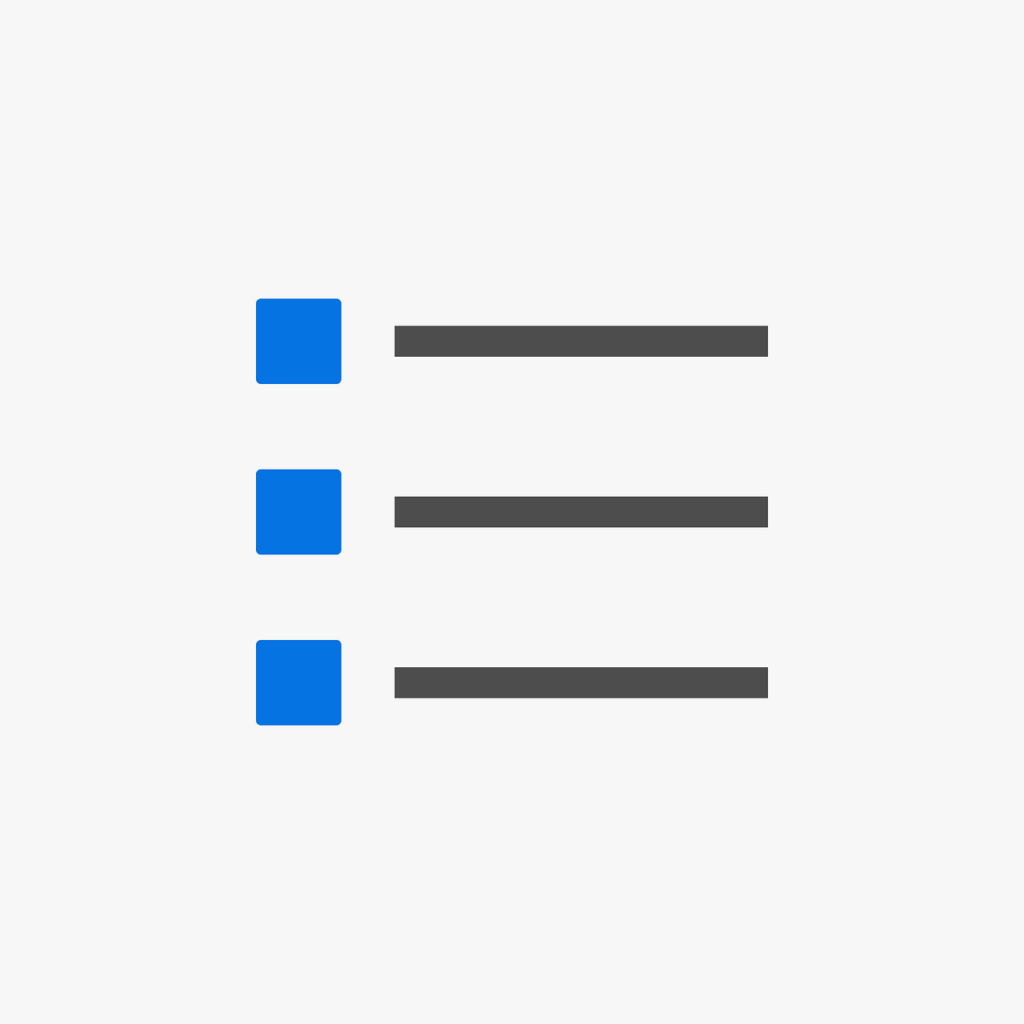
Modifying Lists
It is often necessary to insert or remove elements from a list. Therefore, understanding these operations helps when creating flexible programs. Having the ability to add, remove, or insert ensures that this data structure can handle dynamic content.
Inserting Elements
Sometimes an element must be placed at a specific index, pushing the existing elements forward. This practice keeps the list ordered while accommodating the new item.
Example:
- Suppose there is a list myList ← [5, 6, 7, 8].
- Inserting 9 at index 2:
- The list starts as [5, 6, 7, 8].
- Insert 9 into index 2, pushing the old element at index 2 forward.
- The final list becomes [5, 9, 6, 7, 8].
Adding Elements to the End
A very common procedure is adding a new element to the end. This is similar to appending an item at the bottom of a written list. As a result, it grows in size.
Example:
Code Snippet:
fruits ← ["apple", "banana", "cherry"]
APPEND(fruits, "pineapple")
Step-by-Step:
- Create an empty list called fruits and populate it with three unique fruits.
- The operation APPEND puts “pineapple” at the end.
- The list now becomes [“apple”, “banana”, “cherry”, “pineapple”]
Removing Elements
Removing elements lets you delete an item from any location. Once removed, the rest of the list shifts to fill the gap.
Example:
- Starting with numbers ← [“one”, “two”, “three”[
- Remove the element at index 2 with REMOVE(numbers,2):
- The list is [“one”, “two”, “three”].
- After removal, it becomes [“one”, “three”].
- As a result, it now has two elements.
Determining the Length of a List
Knowing how many elements are in the lists is often essential. The length operation quickly retrieves that count. For instance, if students ← [“Ava”, “Ben”, “Cara”], then LENGTH(students) (or an equivalent method) returns 3. Consequently, programmers can use this information to guide loops or to avoid out-of-bounds errors when accessing elements.
Example:
Code Snippet:
nums ← {2, 4, 6, 8}
DISPLAY(LENGTH(nums))
Step-by-Step:
- The list nums stores four integers.
- LENGTH(nums) evaluates to 4.
- Therefore, the output shows 4.
Traversing a List
Traversing means visiting each element in a list, often to perform some action. A complete traversal touches every item, while a partial traversal visits only a portion. This concept is critical, since many algorithms involve looking at each element. In the real-world, consdering searching for your friend’s name in your phone contacts. You might scroll through every name (complete traversal) or stop once you find what you need (partial traversal).
Iterative Statements for Traversal
Iteration statements, such as REPEAT UNTIL loops, allow you to visit every position in the list. Another popular approach is FOR EACH loops, which go through each element without managing indexes manually.
Example Using FOR EACH Loop:
Code Snippet:
fruits ← ["apple", "banana", "cherry"]
FOR EACH item IN fruits
{
DISPLAY(item)
}
Step-by-Step:
- The loop assigns item to each element in fruits successively.
- On the first pass, item = “apple”, which is printed.
- Next, item = “banana”, which is printed.
- Finally, item = “cherry”, which is printed.
Practical Applications of Lists
Lists often appear in algorithms that look for minimums, maximums, or compute sums. These processes depend on traversing or using indexing to compare items. Because these tasks pop up often, mastering them is crucial for problem-solving.
Example: Finding the Maximum Value
One classic algorithm, called a linear search, allows a program to scan through a list to locate the largest or smallest number. This method compares each element in turn to a “current maximum,” making updates as needed.
Step-by-Step Process:
- Set a variable max to the first element in the list.
- Traverse the list from the second element to the end.
- For each element:
- If the current element is larger than max, update max.
- After reaching the end, max holds the highest value.
Key Terms to Know
- Abstract Data Type – A model for data types where the implementation details are hidden, focusing on set operations.
- List – An ordered collection of elements accessible by index.
- Index – The position of an element in a list; generally starts at 0, but starts at 1 in AP® CSP pseudocode.
- List Procedures – Operations performed on a list, including adding, removing, and accessing elements.
- List Traversal – Moving through a list from one element to another, typically using loops.
- Linear Search – An algorithm that checks each element in sequence until the target is found or the end is reached.
- Iteration Statement – A control structure (like a FOR loop) that repeats a set of instructions multiple times.
Use these definitions as a quick study aid and insert them into your notes for easy reference. By understanding these key terms, it becomes simpler to write, read, and debug code that utilizes lists.
Conclusion
Lists are a powerful abstract data type that allow for easy data manipulation and retrieval. By learning about accessing elements, assigning new values, and embedding these techniques in algorithms, students form a strong foundation in computer science. Moreover, understanding how to insert, remove, and traverse them makes it much simpler to handle bigger programming tasks. Ultimately, frequent practice is key: test these procedures and related operations in small code projects to gain confidence.
Sharpen Your Skills for AP® Computer Science Principles
Are you preparing for the AP® Computer Science Principles test? We’ve got you covered! Try our review articles designed to help you confidently tackle real-world AP® Computer Science Principles questions. You’ll find everything you need to succeed, from quick tips to detailed strategies. Start exploring now!
- AP® Computer Science Principles 3.7 Review
- AP® Computer Science Principles 3.8 Review
- AP® Computer Science Principles 3.9 Review
Need help preparing for your AP® Computer Science Principles exam?
Albert has hundreds of AP® Computer Science Principles practice questions and full-length practice tests to try out.