Strings are among the most common data types found in nearly every programming language. They are essential for tasks like displaying text, handling user input, and even storing passwords. Therefore, it is important for anyone interested in coding to ask: what is a string in programming? In simple terms, a string is a sequence of characters such as letters, numbers, or symbols.
Although strings may look straightforward, they hold hidden complexities like immutability (in some languages) and character indexing. As a result, understanding how to create and manipulate strings can make coding projects much smoother. This article explores what is a string in coding, how to work with strings, and how to use string and substring operations effectively. In the end, a solid grasp of these topics will build a strong foundation for AP® Computer Science Principles.
What We Review
What is a String in Programming?
A string in programming is a sequence of characters enclosed within quotation marks. For instance, “Hello” or “1234” are typical examples of strings. Each character in a string has a specific position, starting from index 0 in many languages.
Imagining these as letters on a mailbox lineup can be helpful. Each letter (or character) has a defined spot. Meanwhile, the entire mailbox name is the string itself. Because the string’s characters are often arranged in a particular order, programmers can easily retrieve individual letters. However, certain languages treat strings as immutable, which means they cannot be changed once created.
This concept matters because it explains why certain operations create new strings rather than alter old ones. Understanding these features helps learners see why strings sometimes behave differently from other data structures or variables.
Creating Strings
There are various ways to create strings, but the most common is using quotes around text. In many languages, double quotes or single quotes can define a string. For example, in Python:
name = "Alice"
This places the word “Alice” in the variable called name. Next, it can be printed or manipulated.
Below is a quick step-by-step guide to creating a string in Python:
- Write a variable name so the string has a place to be stored: my_string =
- Follow it with an equals sign to show assignment.
- Enclose the desired text in quotes: “Coding is fun”
- Combine them: my_string = “Coding is fun”
As a result, the string is now ready to be used in your program.
String Operations
Strings are like building blocks in many projects, and operations such as concatenation and character access make them even more flexible.
String Concatenation
String concatenation joins two or more strings end-to-end to form a new string. For example, consider:
greeting = "Hello, "
name = "Alice"
message = greeting + name
- “Hello, “ is stored in the variable greeting.
- “Alice” is stored in name.
- greeting + name combines them into a new string: “Hello, Alice”.
Therefore, concatenation allows programmers to piece together segments of text for messages, reports, or user feedback.
Accessing Characters in a String
It is also helpful to see how to retrieve specific letters. Suppose the variable word = “Computer”. Accessing word[3] in languages that support zero-based indexing would return p, because positions start at zero:
- C (index 0)
- o (index 1)
- m (index 2)
- p (index 3)
As a result, direct indexing helps programmers handle parts of a string for tasks like spelling checks or password validation.
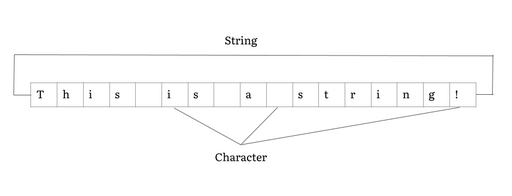
Understanding Substrings
A substring is part of an existing string. Think about how a clip from a full movie still contains the main content but is shorter. For example, if the original string is “Programming”, a substring might be “gram” from characters four to seven.
Substrings are valuable for scanning data within texts, trimming unnecessary spaces, or extracting essential details from user input. Often, languages provide built-in methods like word.substring(startIndex, endIndex) or similar variations. If word = “Programming”, and word[3:7] is used (following Python’s slice notation), the substring would be “gra” (indexes 3, 4, and 5). However, the exact syntax can differ based on the language, so it is worth checking each language’s documentation.
Common String Methods
Strings often come with a set of helpful methods that streamline coding tasks. Here are a few examples used in many programming languages:
- length() or len(): Returns the number of characters in a string.
- find() or indexOf(): Locates the position of a character or substring.
- replace(): Substitutes one substring for another.
- toUpperCase() or upper(): Converts all letters in the string to uppercase.
- toLowerCase() or lower(): Converts all letters in the string to lowercase.
Example: Counting Characters
Consider this snippet in Python:
sentence = "Hello World"
length_of_sentence = len(sentence)
print(length_of_sentence)
- sentence holds “Hello World”.
- len(sentence) counts every character, including the space, resulting in 11.
- The output displays 11 on the screen.
Hence, string methods help automate routine tasks like counting characters, searching for words, or adjusting text formats.
Practical Applications of Strings
Strings take center stage in numerous real-world scenarios. For instance, username and password fields rely heavily on string manipulation to compare input against stored credentials. Similarly, contact forms use strings to collect names, addresses, and messages. As a result, programmers often need to split, join, or search these pieces of text.
In addition, search engines process massive text inputs to find relevant results. Meanwhile, social media platforms rely on string operations for comments, user data, and messages. Therefore, understanding how to handle string and substring operations gives a significant advantage when building interactive applications. By mastering these fundamental skills, aspiring coders gain the ability to tackle larger tasks with greater confidence.
Conclusion
Strings play a vital role in coding and are central to many common processes, making them an integral topic for AP® Computer Science Principles students. These sequences of characters offer powerful ways to handle text, retrieve specific letters, and even combine separate phrases into a single message. In summary, learners should practice creating strings, using string concatenation, and extracting substrings to gain a solid foundation in text-based programming.
Furthermore, exploring common string methods can boost efficiency for tasks like counting, searching, and formatting. The best next step is to try various coding exercises involving text manipulation. With this knowledge in mind, one can confidently navigate more complex programming challenges found in AP® CSP and beyond.
Key Terms to Know
- String – A sequence of characters used to store and represent text.
- Substring – Part of an existing string, identified by specific indices.
- Concatenation – Joining multiple strings to form a new string.
- Index – Position of a character in a string, often starting at zero.
- Immutability – A quality in some languages where a string cannot be changed once created.
- Length – The count of all characters in a string, including spaces.
Sharpen Your Skills for AP® Computer Science Principles
Are you preparing for the AP® Computer Science Principles test? We’ve got you covered! Try our review articles designed to help you confidently tackle real-world AP® Computer Science Principles questions. You’ll find everything you need to succeed, from quick tips to detailed strategies. Start exploring now!
- AP® Computer Science Principles 3.1 Review
- AP® Computer Science Principles 3.2 Review
- AP® Computer Science Principles 3.3 Review
Need help preparing for your AP® Computer Science Principles exam?
Albert has hundreds of AP® Computer Science Principles practice questions and full-length practice tests to try out.